Do you have a large, complex web application that is difficult to maintain and update? If so, you may want to consider using microfrontends. Microfrontends are a new architectural pattern that can help you to break down your application into smaller, independent parts. This can make it easier to manage and maintain, and it can also lead to a more innovative and creative user experience.
Here are some of the problems that microfrontends can solve :
- Complexity : Large, monolithic web applications can be difficult to manage and maintain. They can be difficult to understand, update, and test. Microfrontends can help to break down these applications into smaller, more manageable pieces. This can make it easier to understand the application, update individual components, and test the application as a whole.
- Scalability: Microfrontends can be scaled up or down independently, which can help to improve performance and scalability. If one microfrontend is experiencing high traffic, it can be scaled up without affecting the other microfrontends. This can help to ensure that the application is always performant, even under heavy load.
- Maintenance: Microfrontends can be updated or fixed without affecting the rest of the application. This can save time and resources, and it can also reduce the risk of introducing new bugs. If a bug is found in one microfrontend, it can be fixed without having to update the entire application. This can help to keep the application up to date and bug-free.
- Innovation: Microfrontends can be developed using different technologies and frameworks, which can lead to a more innovative and creative user experience. For example, one microfrontend could be developed using React, while another microfrontend could be developed using Angular. This can give developers more freedom to choose the technologies that are best suited for each microfrontend. It can also lead to a more personalized and engaging user experience, as each microfrontend can be tailored to the specific needs of the user.
In this blog, we’ll be using Single SPA to implement microfrontends. It is a JavaScript framework that allows you to build a single-page application (SPA) that consists of multiple independent microfrontends. Microfrontends are small, self-contained applications that can be developed and maintained independently. This makes it easier to manage large, complex web applications.
Single spa is a good choice for implementing microfrontends because it provides a number of benefits, including:
- Single code base: Single spa uses a single code base for the entire application, including all of the microfrontends. This can save time and resources, and it can also make it easier to manage and update the application.
- Independent development: Microfrontends can be developed independently of each other, which can improve collaboration and communication between teams. This can lead to faster development times and a better user experience.
- Lazy loading: Single spa supports lazy loading, which means that microfrontends are only loaded when they are needed. This can improve performance and reduce the initial load time of the application.
- Shared state: Shared state: Single spa supports shared state, which means that microfrontends can share data with each other. This can be useful for creating a more cohesive user experience.
- Flexible routing: Single spa supports flexible routing, which means that you can define custom routes for each microfrontend. This can give users more control over how they navigate the application.
Overall, single spa is a powerful framework that can help you to implement microfrontends in a way that is efficient, scalable, and flexible. Here are some additional reasons why you might want to use single spa to implement microfrontends :
- Single spa is a mature framework with a large community of users and developers. This means that there are plenty of resources available to help you get started with single spa, and you can be confident that the framework will continue to be supported in the future
- Single spa is easy to use. The framework has a simple API that is easy to learn and use. This makes it a good choice for developers who are new to microfrontends.
- Single spa is flexible. The framework allows you to customize the way that microfrontends are loaded and routed. This gives you a lot of control over the user experience.
Here is a step-by-step guide on how to implement a microfrontend using single spa :
- Create an empty directory and create a sub directory called micro-root.
- Run npm init to initialize an empty package.json.
- Run the following to add the serve command:
npm i --save-dev serve
- Add start command in package.json and type as module:
"start": "serve -s -l 4200", "type": "module"
- Create an index.html file and paste the following into it:
The above HTML code imports the single-spa library, which is used to load microfrontends. It also imports two microfrontends: micro-body and micro-root. The micro-body microfrontend is loaded from the URL http://localhost:5434/main.js, and the micro-root microfrontend is loaded from the file ./public/index.js.
When the browser loads the HTML page, it will first load the single-spa library. Once the single-spa library has loaded, the browser will load the .micro-body microfrontend. The .micro-body microfrontend will then load the micro-root microfrontend.
The micro-root microfrontend is the root of the application. It will then load any other microfrontends that are needed by the application.
This is a basic example of how to implement a single-spa application with two microfrontends. There are many other ways to do it, and the best approach will vary depending on the specific needs of your application. - Add a file called index.js inside the micro-root folder and add the following code into it:
import { registerApplication, start } from 'single-spa'; registerApplication( 'micro-body', () => System.import("micro-body"), (location) => { return true; } ); start();
The above code registers a microfrontend called micro-body with single-spa. The microfrontend is loaded from the URL micro-body and is always active. The code then starts the single-spa application.
We have registered an application in this file. We will be adding the application later in the tutorial. - Run this command to install rollup. It is a JavaScript bundler that can be used to create small, efficient, and modular code.
npm i --save-dev rollup
- Create a file called rollup.config.js inside the micro-root directory and paste the following into it:
export default { external: ["single-spa"], input: ["index.js"], output: [ { dir: "public", format: "system", sourcemap: true } ] };
Here, we are telling rollup that single-spa will be added externally. And we are instructing it to build the index.js file and will put it into a folder called public. This was the reason why we added "type":"module" to package.json. We are telling rollup that we need to build the project as modules.
Follow these steps to create the micro-body application:
- Create an empty angular application from the root of the project using this command:
ng new micro-body - Switch to micro-body directory by running cd micro-body and let's give this application support for microfrontend. To do that, run the following command:
ng add single-spa-angular --project micro-body
Remember to give port as 5434 . - Open micro-body/src/app/app.component.html and add the following code:
- Open micro-body/src/main.single-spa.ts file and remove the following:
import { environment } from './environments/environment';
and add this:
const environment = { production: false }
- Run the following command to serve the application so that it can be integrated with micro-root:
npm run serve:single-spa:micro-body
- If you are getting an error regarding a missing node package run npm install to install any missing dependencies, open another terminal with the micro-root folder and run the following command:
npm run serve
You should see the following output:
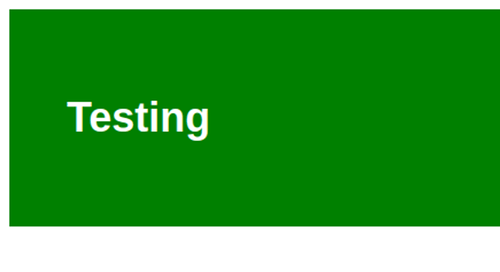
You can follow the above steps to add more applications.
Real-world applications that use single spa to implement microfrontends
- Adobe Experience Manager: Adobe Experience Manager is a web content management system (CMS) that uses single spa to implement microfrontends. This allows Adobe Experience Manager to be more modular and scalable, and it also makes it easier to develop and maintain the application. Spotify: Spotify uses single spa to implement microfrontends for its web player. This allows Spotify to update the web player without having to update the entire application.
- The Guardian: The Guardian uses single spa to implement microfrontends for its website. This allows The Guardian to update different parts of the website without having to update the entire website.
- Atlassian: Atlassian uses single spa to implement microfrontends for its Jira and Confluence products. This allows Atlassian to develop and maintain the products more efficiently.
- Gitlab: Gitlab uses single spa to implement microfrontends for its code review and hosting platform. This allows Gitlab to be more modular and scalable, and it also makes it easier to develop and maintain the platform.
These are just a few examples of real-world applications that use single spa to implement microfrontends. Single spa is a popular choice for implementing microfrontends because it offers a number of benefits, including:
- Modularity: Single spa allows you to develop your application as a collection of independent microfrontends. This can make it easier to develop and maintain the application, and it can also make it easier to update different parts of the application without having to update the entire application.
- Scalability: Single spa can be scaled to support a large number of microfrontends. This makes it a good choice for applications that need to be able to handle a lot of traffic.
- Performance: Single spa can improve the performance of your application by reducing the number of requests that need to be made to the server. This is because single spa only loads the microfrontends that are needed by the user.
While Single spa is a powerful framework, it can also be complex to get started with. It is important to be aware of the challenges before you start using single spa.
Here are some of the challenges of using single spa:
- Complexity: Single spa can be complex to get started with. There are a lot of moving parts, and it can be difficult to understand how everything fits together.
- Dependencies: Single spa relies on a number of third-party dependencies. These dependencies can be updated frequently, and it can be difficult to keep up with the latest versions.
- Debugging: Debugging single spa applications can be difficult. The application is made up of a number of independent microfrontends, and it can be difficult to track down the source of a problem.
- Performance: Single spa can have a negative impact on performance if it is not implemented correctly. The application can become slow and unresponsive if the microfrontends are not loaded efficiently.
Despite these challenges, single spa is a powerful framework that can be used to build great applications. If you are willing to put in the time to learn the framework, you can read the benefits of modularity, scalability, and performance.
Here are some tips for overcoming the challenges of using single spa:
- Start small: Don't try to build a large single spa application as your first project. Start with a small application and gradually add more microfrontends as you become more familiar with the framework.
- Use a good development tool: There are a number of development tools that can help you to manage single spa applications. These tools can help you to debug your application, manage your dependencies, and deploy your application to production.
- Get help from the community: There is a large and active community of single spa developers. There are a number of resources available to help you learn the framework and overcome challenges.
If you are considering using single spa to build your next application, I encourage you to do your research and weigh the pros and cons.
I hope this blog has been helpful. Thank you for reading!!